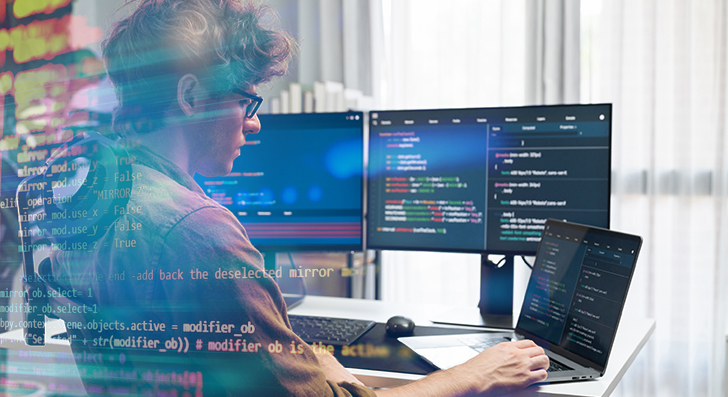
Scalability indicates your software can take care of development—extra people, far more information, and much more traffic—without the need of breaking. Being a developer, developing with scalability in your mind will save time and anxiety later. Below’s a clear and simple guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability isn't anything you bolt on later—it ought to be part of the plan from the start. Many apps fall short every time they expand fast due to the fact the first design and style can’t tackle the extra load. For a developer, you must think early about how your process will behave under pressure.
Commence by building your architecture to become versatile. Avoid monolithic codebases in which every thing is tightly linked. In its place, use modular style or microservices. These designs crack your application into smaller, impartial sections. Each and every module or assistance can scale By itself with out affecting The full process.
Also, think about your database from day one particular. Will it require to deal with 1,000,000 people or simply just a hundred? Choose the correct sort—relational or NoSQL—based upon how your details will expand. Prepare for sharding, indexing, and backups early, Even when you don’t will need them nonetheless.
Another essential stage is to prevent hardcoding assumptions. Don’t compose code that only performs less than current conditions. Consider what would occur In case your user base doubled tomorrow. Would your app crash? Would the databases decelerate?
Use style patterns that assistance scaling, like message queues or event-driven systems. These help your application tackle extra requests without the need of obtaining overloaded.
Whenever you Develop with scalability in mind, you're not just preparing for fulfillment—you might be lessening future headaches. A effectively-planned program is easier to maintain, adapt, and mature. It’s superior to get ready early than to rebuild later on.
Use the proper Database
Selecting the right databases is often a crucial Portion of building scalable applications. Not all databases are crafted precisely the same, and using the wrong one can gradual you down or maybe lead to failures as your app grows.
Get started by comprehension your info. Can it be remarkably structured, like rows inside a desk? If Certainly, a relational databases like PostgreSQL or MySQL is a good healthy. These are generally powerful with interactions, transactions, and consistency. In addition they assistance scaling procedures like go through replicas, indexing, and partitioning to deal with extra targeted traffic and information.
If the information is a lot more flexible—like consumer exercise logs, item catalogs, or files—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing big volumes of unstructured or semi-structured facts and may scale horizontally additional effortlessly.
Also, take into account your read and compose patterns. Do you think you're accomplishing plenty of reads with less writes? Use caching and skim replicas. Are you handling a weighty generate load? Consider databases that could deal with large produce throughput, or even occasion-based mostly facts storage units like Apache Kafka (for temporary information streams).
It’s also sensible to Assume in advance. You may not want State-of-the-art scaling options now, but deciding on a database that supports them means you won’t require to switch later.
Use indexing to speed up queries. Stay away from avoidable joins. Normalize or denormalize your data based on your access patterns. And usually check database functionality while you expand.
In a nutshell, the best databases will depend on your application’s framework, pace wants, And the way you anticipate it to develop. Consider time to pick sensibly—it’ll help you save a lot of trouble afterwards.
Enhance Code and Queries
Rapidly code is vital to scalability. As your app grows, every compact hold off adds up. Poorly penned code or unoptimized queries can slow down general performance and overload your process. That’s why it’s crucial that you Construct effective logic from the beginning.
Start out by composing thoroughly clean, simple code. Stay clear of repeating logic and remove something needless. Don’t choose the most elaborate Resolution if a simple a person will work. Maintain your capabilities limited, focused, and straightforward to test. Use profiling applications to locate bottlenecks—sites in which your code takes far too extended to operate or employs too much memory.
Upcoming, take a look at your databases queries. These frequently gradual points down greater than the code alone. Make certain Just about every query only asks for the information you truly want. Avoid Pick *, which fetches everything, and alternatively find precise fields. Use indexes to speed up lookups. And keep away from doing too many joins, In particular across huge tables.
When you discover precisely the same details getting asked for many times, use caching. Keep the effects temporarily making use of instruments like Redis or Memcached so you don’t really need to repeat highly-priced functions.
Also, batch your database operations once you can. In place of updating a row one after the other, update them in groups. This cuts down on overhead and can make your application extra efficient.
Remember to check with massive datasets. Code and queries that get the job done great with a hundred records may crash after they have to manage one million.
In short, scalable apps are fast apps. Keep your code tight, your queries lean, and use caching when required. These actions aid your application remain easy and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to manage far more end users plus much more website traffic. If anything goes by just one server, it can promptly turn into a bottleneck. That’s the place load balancing and caching are available in. These two tools aid keep your app speedy, secure, and scalable.
Load balancing spreads incoming website traffic across several servers. As opposed to 1 server performing all the do the job, the load balancer routes people to diverse servers depending on availability. This means no single server receives overloaded. If one particular server goes down, the load balancer can ship traffic to the Many others. Instruments like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing information quickly so it could be reused rapidly. When buyers ask for precisely the same info all over again—like a product page or maybe a profile—you don’t must fetch it from the databases each time. You could serve it within the cache.
There are 2 common sorts of caching:
1. Server-facet caching (like Redis or Memcached) merchants data in memory for rapidly access.
2. Shopper-aspect caching (like browser caching or CDN caching) suppliers static information near the user.
Caching lowers databases load, enhances velocity, and tends to make your app far more efficient.
Use caching for things that don’t transform frequently. And normally ensure your cache is current when information does adjust.
In short, load balancing and caching are straightforward but impressive resources. Collectively, they assist your app cope with more consumers, continue to be fast, and Recuperate from challenges. If you propose to grow, you may need both equally.
Use Cloud and Container Tools
To construct scalable apps, you require applications that let your app improve easily. That’s exactly where cloud platforms and containers can be found in. They provide you adaptability, cut down set up time, and make scaling A lot smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and expert services as you would like them. You don’t have to purchase hardware or guess foreseeable future ability. When website traffic boosts, you could increase extra means with just some clicks or quickly applying vehicle-scaling. When targeted visitors drops, you could scale down to economize.
These platforms also present expert services like managed databases, storage, load balancing, and protection instruments. It is possible to deal with making your application as an alternative to handling read more infrastructure.
Containers are An additional crucial Instrument. A container offers your application and almost everything it has to run—code, libraries, configurations—into just one unit. This makes it quick to maneuver your app among environments, from your notebook on the cloud, without having surprises. Docker is the most popular Software for this.
Whenever your app takes advantage of a number of containers, resources like Kubernetes help you regulate them. Kubernetes handles deployment, scaling, and recovery. If one aspect of the application crashes, it restarts it mechanically.
Containers also allow it to be simple to different areas of your application into companies. You are able to update or scale pieces independently, which can be perfect for functionality and reliability.
In a nutshell, using cloud and container instruments indicates you may scale quick, deploy quickly, and recover promptly when issues transpire. If you would like your application to expand without the need of limitations, start out using these equipment early. They help you save time, decrease possibility, and help you remain centered on building, not fixing.
Keep an eye on Everything
Should you don’t watch your software, you won’t know when items go Erroneous. Monitoring will help the thing is how your application is performing, spot troubles early, and make superior decisions as your app grows. It’s a essential Component of building scalable methods.
Commence by tracking standard metrics like CPU use, memory, disk House, and reaction time. These tell you how your servers and solutions are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you collect and visualize this info.
Don’t just check your servers—keep an eye on your application way too. Control just how long it will require for people to load internet pages, how frequently glitches transpire, and wherever they manifest. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Put in place alerts for critical troubles. By way of example, When your response time goes earlier mentioned a limit or even a support goes down, you need to get notified instantly. This assists you fix challenges rapid, generally ahead of consumers even discover.
Checking is likewise valuable once you make modifications. If you deploy a completely new element and see a spike in errors or slowdowns, you may roll it back again prior to it leads to real problems.
As your app grows, traffic and facts boost. Without checking, you’ll skip indications of difficulties till it’s far too late. But with the correct tools in position, you stay on top of things.
In short, checking assists you maintain your app trusted and scalable. It’s not just about recognizing failures—it’s about comprehending your process and ensuring it really works effectively, even stressed.
Last Views
Scalability isn’t just for massive companies. Even modest applications need to have a strong foundation. By coming up with cautiously, optimizing correctly, and using the proper applications, you'll be able to Make apps that expand effortlessly with out breaking under pressure. Get started little, Consider big, and Construct clever.